Sessions using Golang and Redis
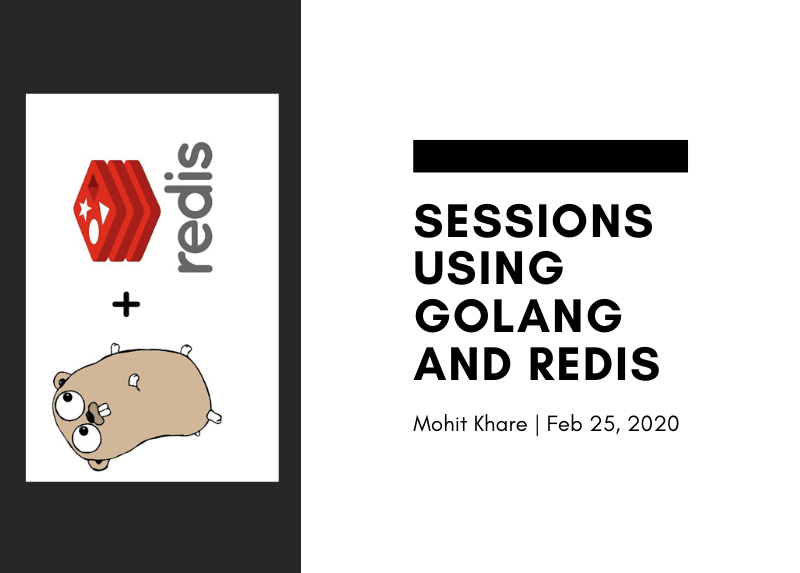
Get latest articles directly in your inbox
In most of the applications/MVP you generally start the implementation with a login/signup feature. With some DB interactions this is quite easy.
But, you don’t want to make user login again and again whenever he opens a new page on your site. You must have noticed on sites like Facebook, Gmail, Twitter — you don’t have to do a login again, rather you are somehow authenticated and you can see the desired home page.
This automatic authentication which persists all over the site is done with the help of cookies and sessions. Let’s understand them one by one.
What are cookies?
Cookies are text files stored on the client machine(eg. web browser) which are useful for tracking purpose. It basically stores some information (which can be key value pair). These values are used by server to perform some actions. It allows developers to easily perform long-term user recognition.
Some useful links on cookies —
- https://developer.mozilla.org/en-US/docs/Web/HTTP/Cookies
- https://medium.com/@endan/working-with-http-cookies-d72f1695a913
What are sessions?
A session can be defined as a server-side storage of information that is desired to persist throughout the user’s interaction with the web site or web application.You can pass info via url parameters too, but mostly you don’t want to expose some fields on client side.
Some useful links on sessions —
- https://stackoverflow.com/questions/3804209/what-are-sessions-how-do-they-work
- Detailed explanation on sessions
Theory
Now, you got the basic idea about cookies and sessions. Let’s now ponder over how we should implement this. Essentially you want to save some cookie on client side, whose value acts as a identifier on server side where you can authenticate user.
Implementation
We will use http cookies and Redis as data store, which will remove old stored sessions after they expire. You can use this for Redis Client
Here we first generate a sessionID
which is a random string. We then add cookie for sessionID via context. You can also add it via http.Request
in your handler.
Then, we add a sessionUser which is a struct —
type SessionUserClient struct {
UserID uint
Authenticated bool
}
// You can add fields like "Visitor count", etc according to usecase
Now, let’s persist the cookie value in our redis and store the SessionUser
object. Here, I set the expiry time in cookie and redis for 180 seconds. You can change it, (say 24 hours)
How to retrieve and check for cookies?
Once a user hits a URL which requires authentication —
- We check if we received a cookie
- Fetch the cookie value
- Check in redis if the key exists.
- Fetch the user and check if
Authenticated
param is set totrue
Since, you want to check session over multiple page, adding a middleware seems a better option here. Read about implementing middleware here.
Time to Test
- Create a /signup route, where you create a session for user.
- Create another /profile route, add session auth middleware to this route. You’ll see you can access this page if you are logged in.
- To refresh token, use /signin page. Create new sessionID there and make a new entry to redis.
Now, you can add sessions to your new project. 😎
Liked the article? Consider supporting me ☕️
I hope you learned something new. Feel free to suggest improvements ✔️
I share regular updates and resources on Twitter. Let’s connect!
Keep exploring 🔎 Keep learning 🚀
Liked the content? Do support :)