Running periodic background tasks in Golang
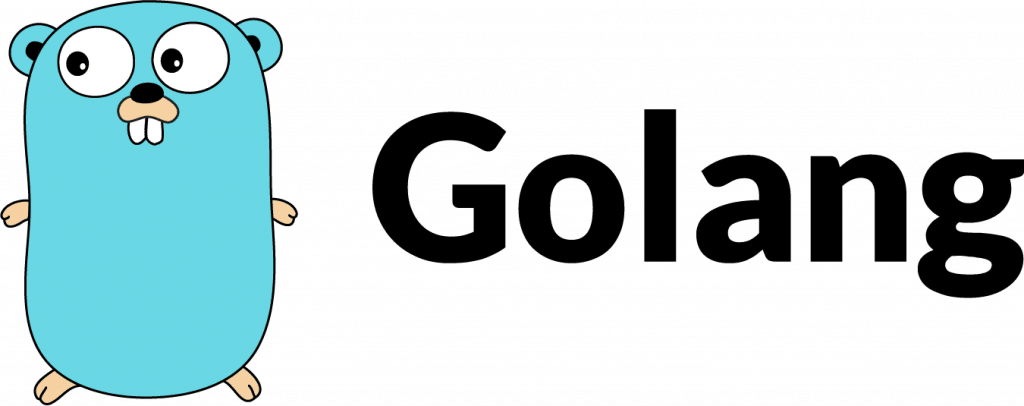
Get latest articles directly in your inbox
Problem - I had to download some files in the background every day on my Golang server. So, let’s break this problem into two parts -
- Run tasks periodically.
- Run tasks in the background.
Run tasks periodically
If you are aware of background tasks, you must have come across/used Linux Cronjob sometime.
But wait, What is Cron?
Cron allows Linux and Unix users to run commands or scripts at a given date and time. You can schedule scripts to be executed periodically. Easy! No? Just a simple Linux command.
Coming back on how do we do this in Go?
After searching for a while, I came across this package gocron. Well, this is what I was looking for. Let’s see how this works!
package main
import (
"fmt"
"github.com/jasonlvhit/gocron"
)
func myTask() {
fmt.Println("This task will run periodically")
}
func executeCronJob() {
gocron.Every(1).Second().Do(myTask)
<- gocron.Start()
}
func main() {
go executeCronJob()
SomeAPICallHandler() // handler which accepts requests and responds
}
Okay, this is as simple as it looks. I created a myTask() function which will do some task. Then in my executeCronJob(), I start the cron job and set it for every second.
You can also set it for every minute, hour, day, week, etc.
gocron.Every(1).Minute().Do(myTask) // Every Minute
gocron.Every(2).Hours().Do(myTask) // Every 2 Hours
gocron.Every(3).Days().Do(myTask) // Every 3 Days
gocron.Every(4).Weeks().Do(myTask) // Every 4 Weeks
You get the gist, check out the documentation of this awesome package to explore this more and use it as you want.
Run tasks in the background
Coming to the only line of our main, we see -
go executeCronJob()
Okay, you must be asking is it this simple to run a task in the background?
The answer is YES.
Basically the executeCronJob() function will run in the background in a goroutine.
What is goroutine ?
A goroutine is a lightweight thread of execution. So, Goroutines are functions that run concurrently with other functions or methods.
Few links on understanding concurrency and goroutines which I found useful and learned from:
- https://medium.com/@matryer/very-basic-concurrency-for-beginners-in-go-663e63c6ba07
- https://golangbot.com/goroutines/
Catch in above code
So, If you are just executing go executeCronJob() in your main. Then, your background tasks won’t run since your main has exited and the program has stopped.
Either you need to have a server like in my case which runs continuously thus program doesn’t exit or you need to put your function to sleep so that you can verify your background task is actually running. Add this code to see your background task in action -
time.Sleep(500 * time.Millisecond)
Yay! Now it worked. Feel free to improve the article. I am learning too 😃 Time to Go 🚀
Liked the article? Consider supporting me ☕️
Resources
Books to learn Golang
Popular Go Articles
I hope you learned something new. Feel free to suggest improvements ✔️
I share regular updates and resources on Twitter. Let’s connect!
Keep exploring 🔎 Keep learning 🚀
Liked the content? Do support :)