Learn Maps in Golang (with examples)
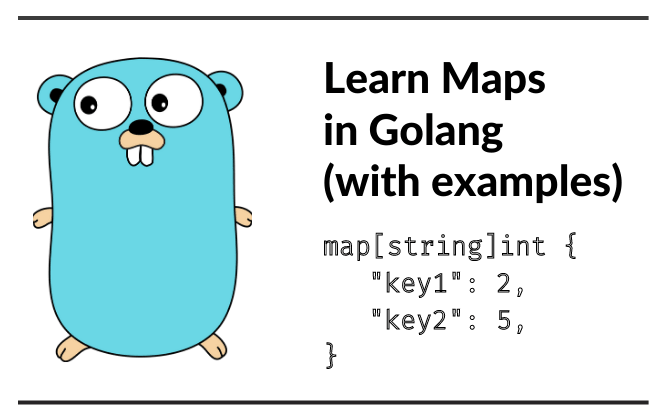
Get latest articles directly in your inbox
In this article, we will be covering the basics of map. We will cover how to create maps, add and remove items, iterating through them and even explore equality in maps. After this, you will be ready to use maps in your Golang programs.
Let’s begin!
What is a map?
A map is basically a collection of key-value pairs. If you are coming from Python, map is basically a dictionary. Maps are used for fast lookups, retrieval, and deletion of data using the keys. Each key in a map is unique and acts as a identifier to retrieve values. In Golang, maps are a built-in datatype and they are unordered.
Creating maps
Creating maps in go is pretty straightforward. Here we see how to declare and initialise maps.
Declaration
// Declaring a map
var name_of_map map[key_datatype]value_datatype{}
Here’s an example where we create a map with string
keys and integer
value.
// userAge is a map which stores age of person.
// Name as key and Age as value.
var userAge map[string]int{}
Go provides another way to declare maps with the make
function. We create similar map as before but with make
command.
// Create map using make keyword
make(map[key_datatype]value_datatype)
// Example
userAge := make(map[string]int)
// fmt.Println(userAge) -> map[]
Initialisation
Now, we can initialise a key value pair in userAge
map simply by separating with commas.
package main
import (
“fmt”
)
func main() {
var userAge = map[string]int{
“mohit”: 24,
“nidhi”: 21,
}
fmt.Println(userAge)
}
Output for above code will be -
map[mohit:24 nidhi:21]
Adding key-value to map
We can add kay-value pair to map using -
your_map_name[key] = value
Here we declare a map and then add 2 key-value pair to it.
package main
import (
“fmt”
)
func main() {
// Declare a map
userAge := make(map[string]int)
// Add key “mohit” with value 24
userAge[“mohit”] = 24
userAge[“nidhi”] = 21
fmt.Println(userAge)
}
Output for above code will be same as before -
map[mohit:24 nidhi:21]
We can update a key value pair same way as we add. Example - userAge[“mohit”] = 20
Removing key from map
We can delete a key-value from map using the delete()
function
delete(your_map_name, key_name)
An example for this -
package main
import (
“fmt”
)
func main() {
// Declare a map
userAge := make(map[string]int)
// Add key “mohit” with value 24
userAge[“mohit”] = 24
userAge[“nidhi”] = 21
fmt.Println(“Before delete”, userAge)
delete(userAge, “mohit”)
fmt.Println(“After delete”, userAge)
}
Retrieving value from a key
Once we add, we will also want to retrieve the value. This can be done with your_map_name[key]
Let’s extract value to key mohit
from userAge
map from before.
package main
import (
“fmt”
)
func main() {
var userAge = map[string]int{
“mohit”: 24,
“nidhi”: 21,
}
fmt.Println(userAge[“mohit”])
// Output - 24
}
Check if key exists?
We learned how to get value but what if try to retrieve a key which doesn’t exist in the map. In that case, we get zero value of the map. Check the example -
package main
import (
“fmt”
)
func main() {
var userAge = map[string]int{
“mohit”: 24,
}
fmt.Println(“Zero value when key not present”, userAge[“nidhi”])
// Check if key exists?
value, isPresent := userAge[“mohit”]
if isPresent {
fmt.Println(“Key present: mohit’s age - “, value)
} else {
fmt.Println(“Key not present”)
}
_, ok := userAge[“nidhi”]
if ok {
fmt.Println(“Key present”, value)
} else {
fmt.Println(“Key not found”)
}
}
Output for the code above -
Zero value when key not present 0
Key present - mohit’s age - 24
Key not found
Iterating through maps
We can use range keyword to loop through a map. range
returns two variables - key
and value
( obviously you can use any variable). Iteration order over maps is random and unstable i.e it may or may not be different when you run the same program multiple times. Details here!
Although you can sort the map by using sort
function.
package main
import (
“fmt”
)
func main() {
var userAge = map[string]int{
“mohit”: 24,
“nidhi”: 21,
}
for key, value := range userAge {
fmt.Println(key, value)
}
}
// Output
mohit 24
nidhi 21
Check if two maps are equal?
Maps cannot be directly compared using ==
operator. Equality operator only works when both maps are empty. Though you can check if each key has same value in both maps by iterating through them.
package main
import (
“fmt”
)
func main() {
userAge1 := map[string]int{
“mohit”: 24,
}
userAge2 := map[string]int{
“mohit”: 24,
}
if userAge1 == userAge2 {
fmt.Println(“Maps are equal”)
} else {
fmt.Println(“Maps are unequal”)
}
}
The code above gives an error -
invalid operation: userAge1 == userAge2 (map can only be compared to nil)
Bonus on maps
- You can get length of map using
len()
function. Eg.len(your_map)
- Maps are reference types i.e if you change map instance then changes will be reflected wherever that map is used.
- Maps are not thread-safe
- You can clear a map by simply assigning it to empty map. Eg.
your_map = map[string]bool{}
Resources
Books to learn Golang
Awesome! You just learned how to use maps in Go. There is lot more to maps, will be covering more in other tutorials.
Liked the article? Consider supporting me ☕️
I hope you learned something new. Feel free to suggest improvements ✔️
I share regular updates and resources on Twitter. Let’s connect!
Keep exploring 🔎 Keep learning 🚀
Liked the content? Do support :)