Git Branch 101
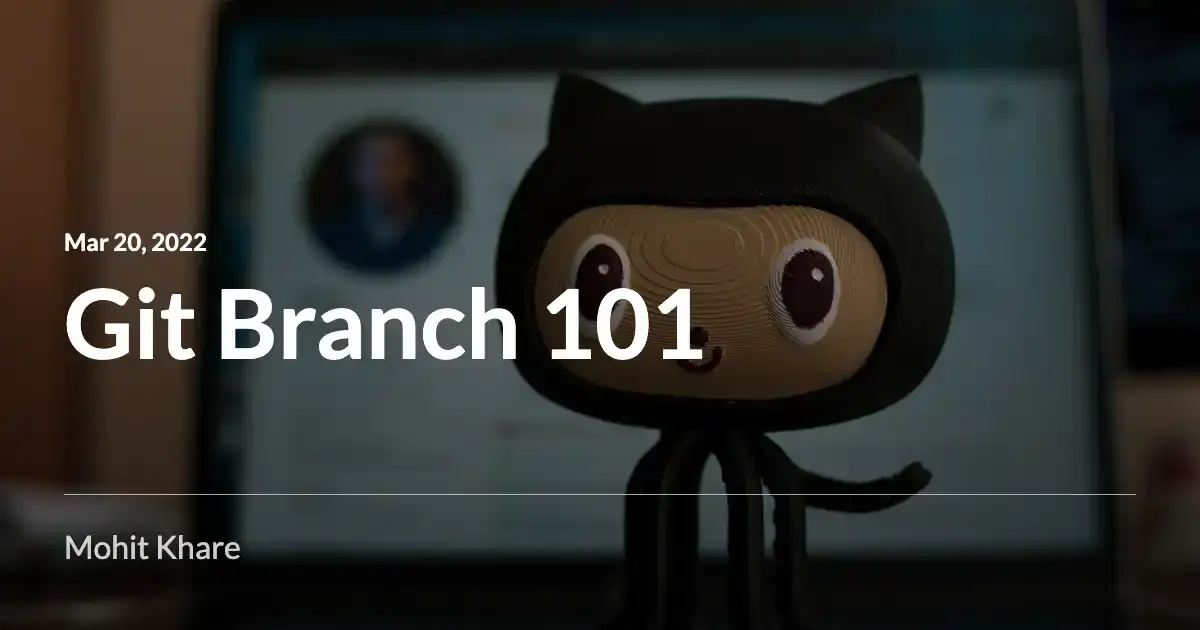
Get latest articles directly in your inbox
There are high chances that you’re using Git or some VCS system in your day-to-day work life. Branching is one of the most common and useful component in version control systems like Git.
You can think of branch like a version of the project that is usually used to build a new feature, fix a bug or perform some code operation on top of the main project. At all times during development, your main codebase is unaware of any changes on a branch till the point the changes are merged. Git provides us a way to create and manage the branches in a project via a set of branch
commands. But before we learn how to use branching, let’s understand why do we need it?
Need of Branching
You might think why would we create a new branch? Why can’t we directly add features to the main project?
Fair enough! We could directly build on the codebase but there are a couple of issues with that. Let’s explore them!
- You don’t want to affect the main codebase. Imagine there’s a bug in your changes and those changes are directly live to customers of your application. Branching essentially adds a barrier for any unstable code to be tested and reviewed before being pushed to main codebase.
- Usually a lot of people are working on a project at the same time. Imagine the pain of managing multiple changes during development cycle. Branching offers a way to work on a new feature without affecting the main codebase thus multiple features can be built in parallel.
- A branch is like a separate path of development. You can edit, delete or update any changes till the point it’s merged. Before changes are pushed to main codebase, you can clean up the testing changes or code smells. This ensures your main branch of development is production ready at all times.
How it works?
In Git, a branch is stored as a reference to a commit. Point to note here is that branch doesn’t store a list of commits. As mentioned earlier think of it as a new path of development where the tip of branch points to some commit on main codebase.
When you initialize git on a project, a master branch is created by default. This is the branch that git provides us for development. As you add changes via commits, your master branch keeps on growing. Your master branch is the “current working version” of your project at all times.
A new branch is like a new working arena where you can add, update or remove existing code. All the commits are part of history of this new branch you created. Eventually once all your changes are done, these changes are merged into the master branch.
The git branch
command provides us a way to manage development on branches. One can create, list, rename, delete and switch branches using these commands. Let’s see these commands in practice.
Firstly we need to setup a project with git for the git branch commands to work.
# create a new directory
mkdir learn-git-branch
cd learn-git-branch
# initialize a git repository
git init
# create a file to work on
touch somefile.txt
# Open your favourite editor and add some text
Lets learn git branch
# Commit your changes on master
git add .
git commit -m "Initial commit"
# Run git branch to verify that master branch is created
git branch
Listing branches
To list out the existing branches, we can directly use the git branch command without any flags. There are also few flags available that we’ll cover here.
# Lists exisiting branches
git branch
# Same as before
git branch --list
# List both remote-tracking branches and local branches
git branch --all
The current branch will be green in color and highlighted with an *
(asterisk). We will be using the list commands in other sections to verify branching operations.
Creating a branch
We can create a new branch using the following command.
git branch <branch-name>
# create branch in our project
git branch new-feature
Now if you run the git branch
command, you should see two branches.
* master
new-feature
Awesome, we create a new branch successfully. But you can see the *
pointing on master branch. This means we created a new branch but our current active branch is still master
. Git branch just creates a branch. To make changes we would need to checkout to that branch.
To create a remote branch, we just need to push our local branch. First define a remote name with URL and then push branch to the remote.
git remote add <remote-name> <git-url>
git push <remote-branch> <branch-name>
Deleting a branch
Deleting a branch is quite straightforward. We can use the -d
flag for that.
git branch -d <branch-name>
# Delete the branch we created
git branch -d new-feature
# Output
Deleted branch new-feature (was 6d0f739).
The commit id in output would be different for you. You can run git log
to see the commit id for the commit we created earlier. The tip of the new-feature
branch was this commit itself.
Let’s run git branch
again to verify if our branch was deleted. Now you should only see the master branch.
* master
Note: -d
flag is a safe operation ie. it only deletes a branch when there are no changes or the branch is fully merged. In other cases this would throw an error. One can still do a force delete on a branch using -D
option.
# Forceful branch delete
git branch -D <branch-name>
To delete a remote branch, the idea is similar to the creating a remote branch. We push changes to remote.
git push -d <remote_name> <branchname>
$ git branch -d <branchname>
Switching a branch
Till now we learned how to list, create and delete a branch. What if we want to switch between branches when working on multiple features. We can do this using the checkout
command.
# We deleted our branch. Create it again. Ignore if branch exists.
git branch new-feature
# command to switch branch
git checkout <branch-name>
# Switch to new-feature branch
git checkout new-feature
# Verify the current branch
git branch
To switch back to master branch we can do git checkout master
.
There’s also a shortcut to create a new branch and switch to the newly created branch.
git checkout -b <branch-name>
Renaming a branch
Git provides us with an option to rename a branch as well.
git branch -m <old-branch> <new-branch>
# Rename our new-feature branch
git branch -m new-feature new-feature-renamed
# Verify if the branch name is updated
git branch
# Output
* master
new-feature-renamed
Merging a branch
We’ll make some changes and create a commit on the newly created branch.
# Switch to new-feature branch
git checkout new-feature
# Make some changes in somefile.txt
# Stage the changes
git add .
# Create a commit
git commit -m "Adding new changes"
Now we are done with changes on the branch and say want to merge this into master. Here is how we can do it using the merge
command.
# Switch to branch where we want the changes to be merged
git checkout master
# Command to merge the branch
git merge <branch-name>
git merge new-feature
Tada 🥳 , the changes are now merged to master. You can verify the commits on master using git log
Resources
Do explore articles on Golang and System Design. You’ll learn something new 💡
Liked the article? Consider supporting me ☕️
I hope you learned something new. Feel free to suggest improvements ✔️
I share regular updates and resources on Twitter. Let’s connect!
Keep exploring 🔎 Keep learning 🚀
Liked the content? Do support :)